Enhancing Android WebView Experiences with Offline Fallback and Smart Performance Cache
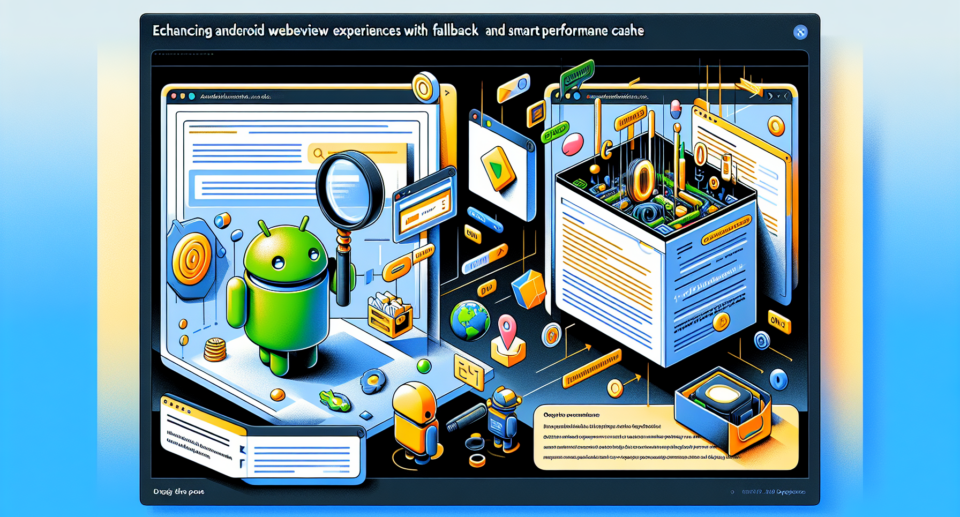
Understanding Android WebView
Android WebView is a powerful component that allows developers to display web content within an application. It bridges the gap between web and native experiences, making it easier to integrate online features into your app. However, challenges such as intermittent connectivity and performance optimization still need addressing.
The Challenge of Offline Access
One significant challenge in using WebView is ensuring that your app remains functional even when offline. Users expect a consistent experience, and losing access to content because of network issues can lead to frustration. By implementing offline fallback mechanisms, you can provide a more resilient user experience.
Implementing Offline Fallback
To enable offline fallback, you need to cache web resources locally. This can be achieved by using Service Workers and caching strategies within your WebView implementation. Here’s a basic approach:
// Register a Service Worker
webView.getSettings().setJavaScriptEnabled(true);
webView.getSettings().setDomStorageEnabled(true);
webView.setWebViewClient(new WebViewClient() {
@Override
public void onPageFinished(WebView view, String url) {
// Register your Service Worker here
view.evaluateJavascript(if ('serviceWorker' in navigator) { navigator.serviceWorker.register('/service-worker.js'); }, null);
}
});
This script ensures that a Service Worker is registered when the page loads. The Service Worker will handle fetching and caching resources, providing offline support.
Smart Performance Cache
To further enhance the WebView experience, implementing a smart performance cache can significantly reduce loading times and improve responsiveness. By caching frequently accessed resources and preloading critical assets, you can create a snappy and fluid user interface.
Optimizing WebView Performance
Here are some tips for optimizing WebView performance:
- Enable Caching: Ensure that caching is enabled in your WebView settings to allow for quicker load times of previously visited pages.
- Preload Resources: Preload essential resources such as images, scripts, and stylesheets to minimize wait times during navigation.
- Use